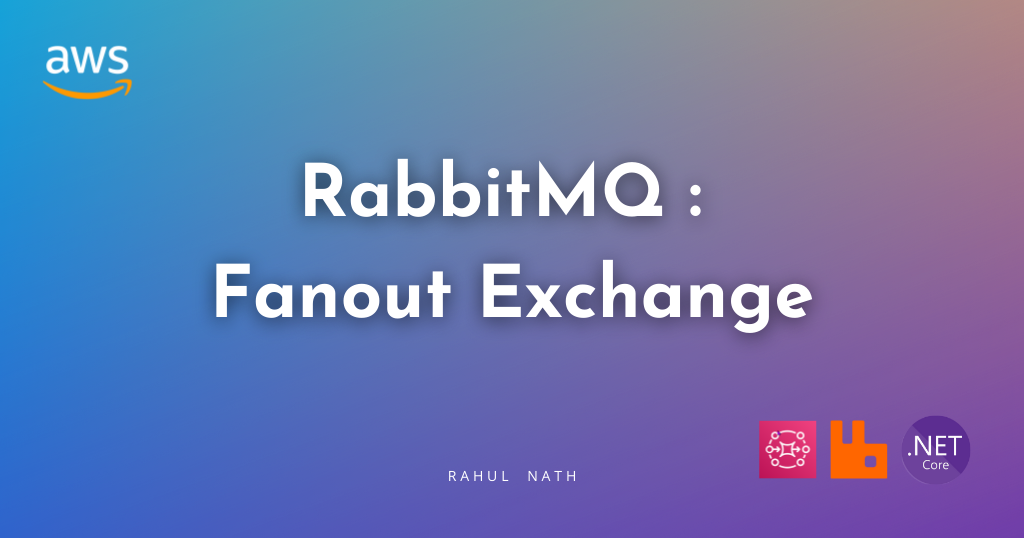
RabbitMQ Fanout Exchange Explained
A Fanout Exchange type in RabbitMQ routes messages to all of the queues bound to it, ignoring the routing key. Let's understand how to set up a Fanout Exchange and how it routes messages to consumers.
Table of Contents
An Exchange in RabbitMQ is a routing mechanism to send messages to queues.
RabbitMQ supports four different Exchange Types.
- Topic Exchange
- Fanout Exchange
- Headers Exchange
- Direct Exchange
In this blog post, let's understand
- RabbitMQ Fanout Exchange
- Routing messages in Fanout Exchange
- Set up a Fanout Exchange from .NET app
I use Amazon MQ, a managed message broker service that supports ActiveMQ and RabbitMQ engine types, to host my RabbitMQ instance. However, you can use one of the various options that RabbitMQ provides to host your instance,
AWS sponsors this article and is part of my RabbitMQ Series.
What is a RabbitMQ Fanout Exchange?
A Fanout Exchange type in RabbitMQ routes messages to all the queues bound to it, ignoring the routing key.
When a new message is published to a Fanout exchange, a copy is delivered to all the queues bound to it.
Fanout exchanges are ideal for broadcasting messages.
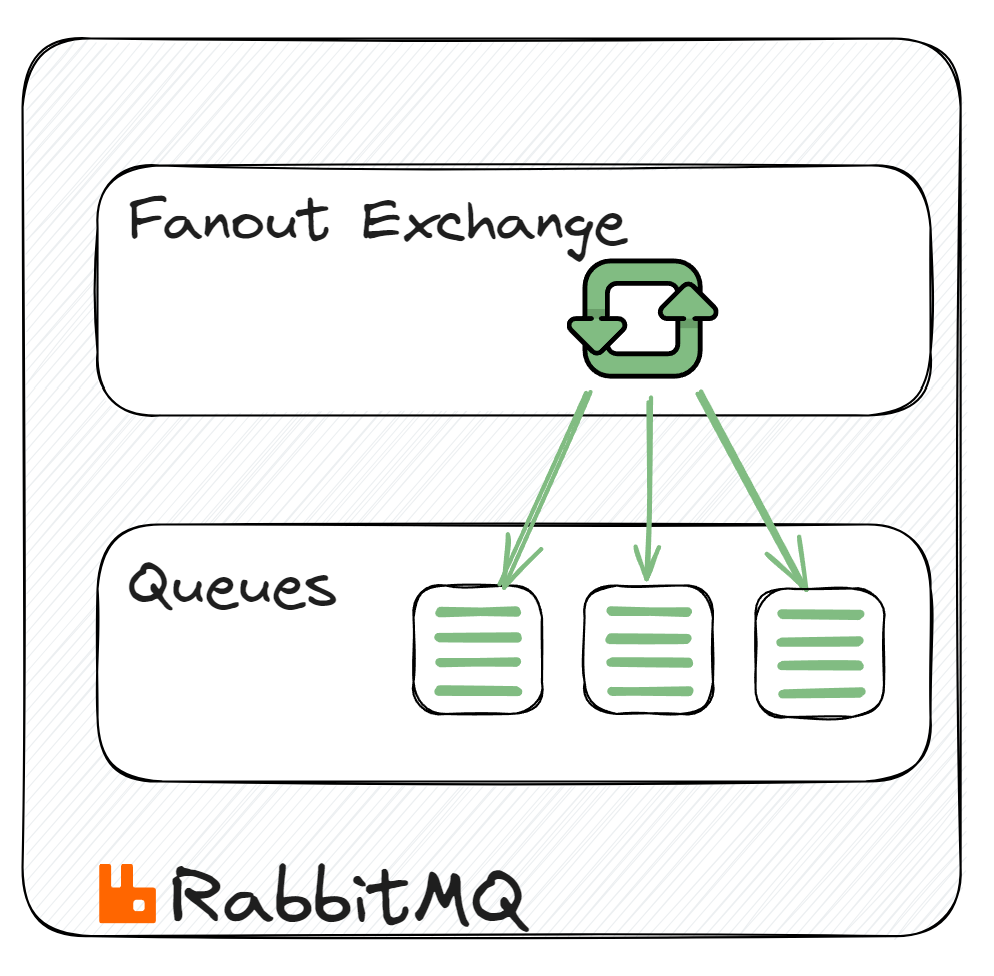
Fanout exchange ignores the routing keys on the messages and any Binding keys if specified.
RabbitMQ Fanout Exchange From .NET
We will use a NuGet package, RabbitMQ.Client, to connect and send/receive messages from RabbitMQ.
If you are new to building RabbitMQ from .NET applications, check out the Getting Started article below.
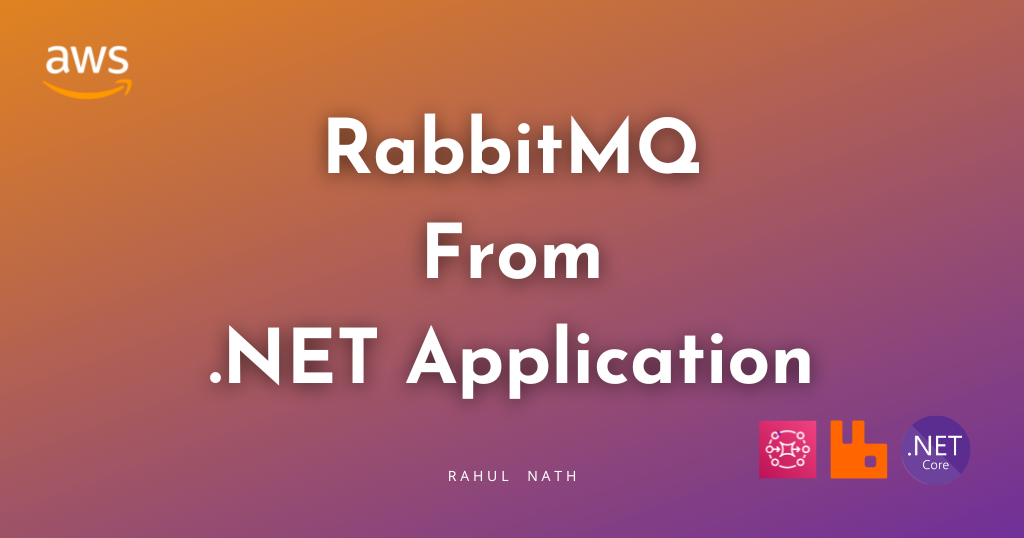
Creating RabbitMQ Fanout Exchange From .NET
Exchanges are created using the Channel.
The ExchangeDeclare
the method takes in an exchange name and its type. Below is an example of creating a RabbitMQ Fanout Exchange in .NET.
var factory = new ConnectionFactory()
{
Uri = new Uri("YOUR RABBIT INSTANCE URI"),
Port = 5671,
UserName = "<USERNAME FROM CONFIGURATION FILE>",
Password = "<PASSWORD FROM CONFIGURATION FILE>"
};
using var connection = factory.CreateConnection();
using var channel = connection.CreateModel();
var exchangeName = "weather_fanout";
channel.ExchangeDeclare(exchangeName, ExchangeType.Fanout);
When sending a message, the sender specifies the Exchange and routing key.
channel.BasicPublish(exchangeName, routingKey, null, body);
When sending to a Fanout Exchange, the routingKey
can be empty as the exchange ignores it.
Set up Binding on Fanout Exchange From .NET
Messages are routed to Queues from Exchanges using the Binding information.
To create a Queue, use the QueueDeclare
method. It will create a new Queue only if one doesn't exist with the same name.
var factory = new ConnectionFactory()
{
Uri = new Uri("YOUR RABBIT INSTANCE URI"),
Port = 5671,
UserName = "<USERNAME FROM CONFIGURATION FILE>",
Password = "<PASSWORD FROM CONFIGURATION FILE>"
};
using var connection = factory.CreateConnection();
using var channel = connection.CreateModel();
var queueName = "nsw";
channel.QueueDeclare(queueName, false, false, false, null);
channel.QueueBind(queueName, "weather_fanout", string.Empty);
The above code sets up a queue nsw
and sets up a binding with the Fanout exchange (weather_fanout
) we created earlier.
You can create multiple queues and bind with the same exchange, if multiple consumers are interested in the messages coming to the Exchange.
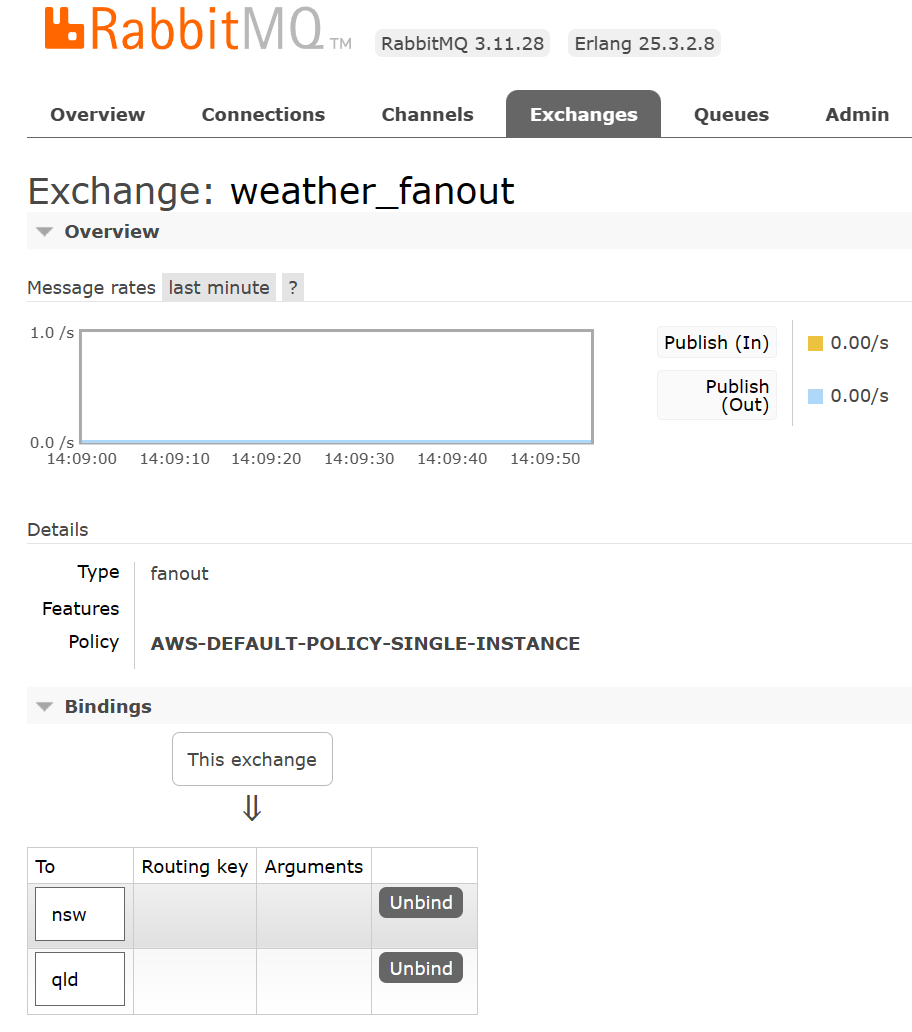
For example, In this case, the weather_fanout
exchange can be used to broadcast critical weather alerts, and all consumers are interested in it. To register other Queues, we can repeat the queue declaration and binding.
var queueName = "qld";
channel.QueueDeclare(queueName, false, false, false, null);
channel.QueueBind(queueName, "weather_fanout", string.Empty);
Any time a message is sent to the Exchange, a copy of it is sent to both qld
and nsw
Queues and the consumers listening on those queues can process the messages.
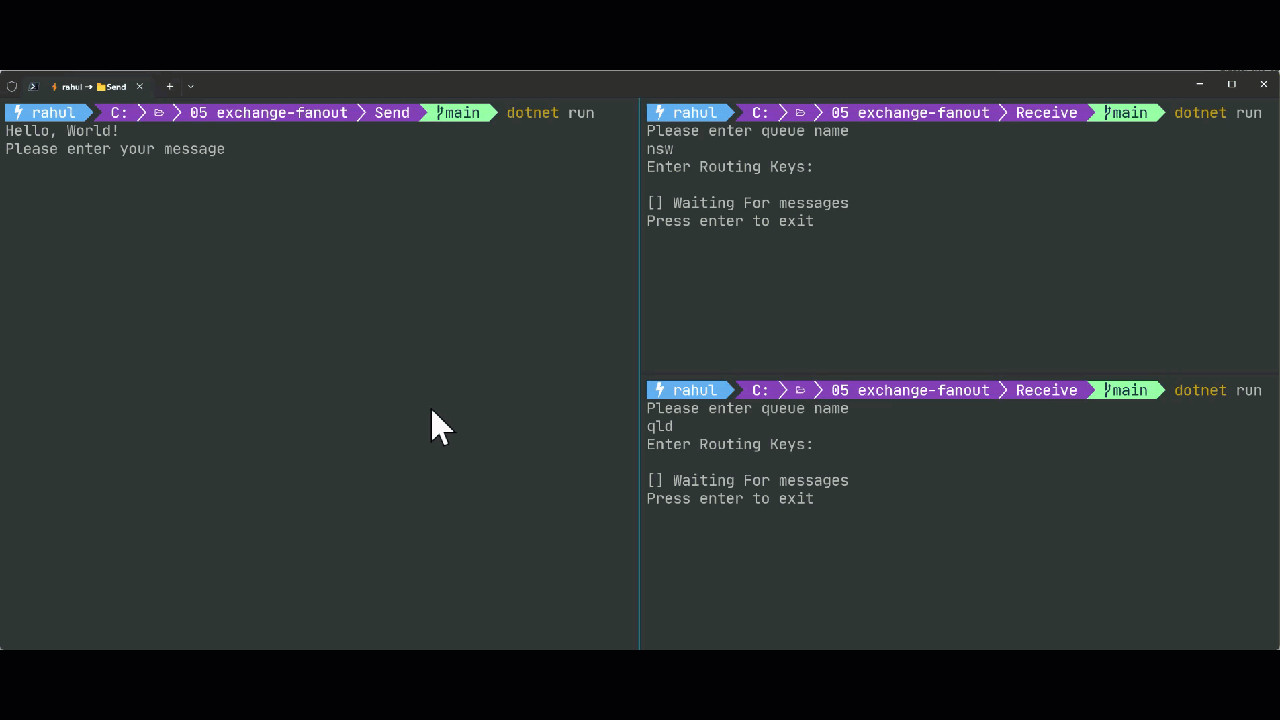
Rahul Nath Newsletter
Join the newsletter to receive the latest updates in your inbox.